Start A New MDB Vite React Application Project
- Forums
- react
- Start A New MDB Vite React Application Project
This Page Contains information about Start A New MDB Vite React Application Project By edw in category react with 0 Replies. [5233], Last Updated: Fri Nov 24, 2023
edw
Wed Nov 22, 2023
0 Comments
124 Visits
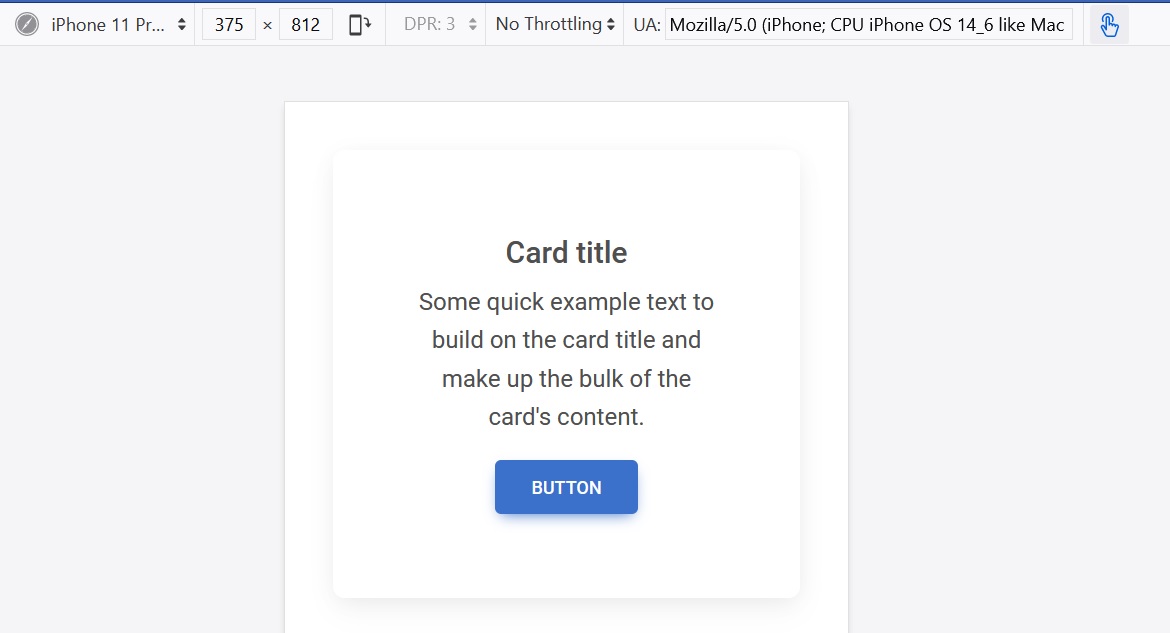
screenshot of the app in mobile mode in browser.
- create a new blank project
$ npm create vite@latest
- Give it a project Name
- Select the platform
$ cd project name
$ npm install
$ npm i mdb-react-ui-kit
$ npm i @fortawesome/fontawesome-free
$ code .
$ code src/App.tsx
- Add imports to App.js:
import 'mdb-react-ui-kit/dist/css/mdb.min.css';
import "@fortawesome/fontawesome-free/css/all.min.css";
$ code index.html
- Add to index.html
<link href="https://fonts.googleapis.com/css?family=Roboto:300,400,500,700&display=swap" rel="stylesheet" />
$ code src/index.css
- Change src/index.css to:
body {
font-family: Roboto, Helvetica, Arial, sans-serif;
}
- start application:
$ npm run dev
- Add authentication: https://www.webune.com/forums/add-authentication-to-react-vite-using-laravel-backend.html
- Download Components from Github:
https://github.com/mdbootstrap/mdb-react-ui-kit
or visit their site for more information:
https://mdbootstrap.com/docs/react/getting-started/installation/
- Create a new Component in src/components/Card.tsx with this BASH command
$ mkdir -p src/components/mdb/; touch
src/components/mdb/Card.tsx; code
src/components/mdb/Card.tsx
- Copy and paste the following code into Card.tsx
-
import {MDBBtn,MDBCard,MDBCardBody,MDBCardText,MDBCardTitle,} from "mdb-react-ui-kit";
function Card() {
return (
<MDBCard>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the
bulk of the card's content.
</MDBCardText>
<MDBBtn>Button</MDBBtn>
</MDBCardBody>
</MDBCard>
);
}
export default Card;
$ code src/App.tsx
- Change App.tsx to the following:
import 'mdb-react-ui-kit/dist/css/mdb.min.css';
import "@fortawesome/fontawesome-free/css/all.min.css";
import Card from './components/mdb/Card';
function App() {
return (
<Card />
)
}
export default App
- http://localhost:5173/